What is scope in java script ?
Scope simply means an area where the program can access a particular variable or a function.
Types of scope –
- Global Scope
- Local Scope/ Functional Scope
- Block Scope
Global Scope
var greeting = 'Hello World!';function greet() {
console.log(greeting);
}// Prints 'Hello World!'
greet();
Local Scope
function greet() {
var greeting = 'Hello World!';
console.log(greeting);
}// Prints 'Hello World!'
greet();
console.log(greeting);
Block Scope
{
let greeting = 'Hello World!';
var lang = 'English';
console.log(greeting); // Prints 'Hello World!'
}// Prints 'English'
console.log(lang);// Uncaught ReferenceError: greeting is not defined
console.log(greeting);
Scope of a variable is directly proportional to its lexical scope
What is scope chain ?
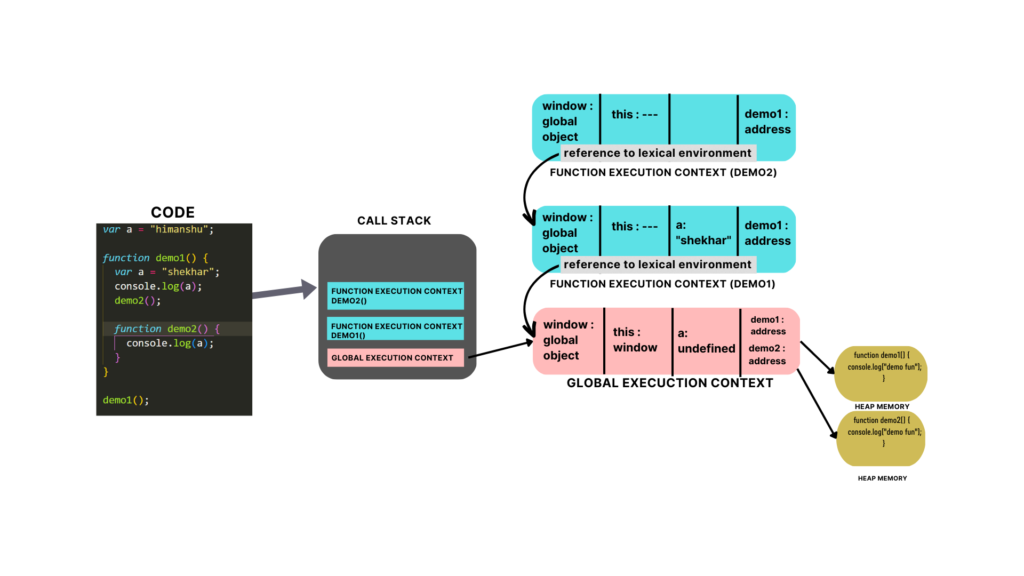
Let understand this with an code –
var a = "himanshu"; function demo1() { var a = "shekhar"; console.log(a); demo2(); function demo2() { console.log(a); } } demo1();
Taking the above example , the js pointer , does not directly jump to global space and find the variable a , instead it follow as specific path –
- first, it search inside demo2() , if not found then ,
- it goes to the outer/parent function , if not found then,
- it searches in the global scope ,
thus forming a chain , known as scope chain.
demo2() scope ---> demo1() scope ---> global scope
What is Lexical Scope ?
Also know as static scope.
Lexical environment of a variable is a combination of - -> local memory and -> parent lexical scope
Lets understand this with an code example –
var a = "himanshu"; function demo1() { var a = "shekhar"; console.log(a); demo2(); function demo2() { console.log(a); } }
Practice –
Question 1 –
const fullName = "Jhon";
function profile() {
const fullName = "Tobi";
console.log(fullName);
function sayName() {
console.log(fullName);
function writeName() {
const fullName = "Shah";
console.log(fullName);
}
writeName();
}
sayName();
}
profile();
Question 2
function showLastName() {
const lastName = "Sofela";
return lastName;
}
// Define another function:
function displayFullName() {
const fullName = "Oluwatobi " + showLastName();
return fullName;
}
// Invoke displayFullName():
console.log(displayFullName());