Lets understand the working with an simple example –
var a = "hello world"; console.log(a); function demo1() { console.log("demo1 fun"); demo2(); } function demo2() { console.log("demo2 fun"); } demo1(); console.log("It is GEC");
Now as soon as js engine detects a java script code, it creates an execution context.
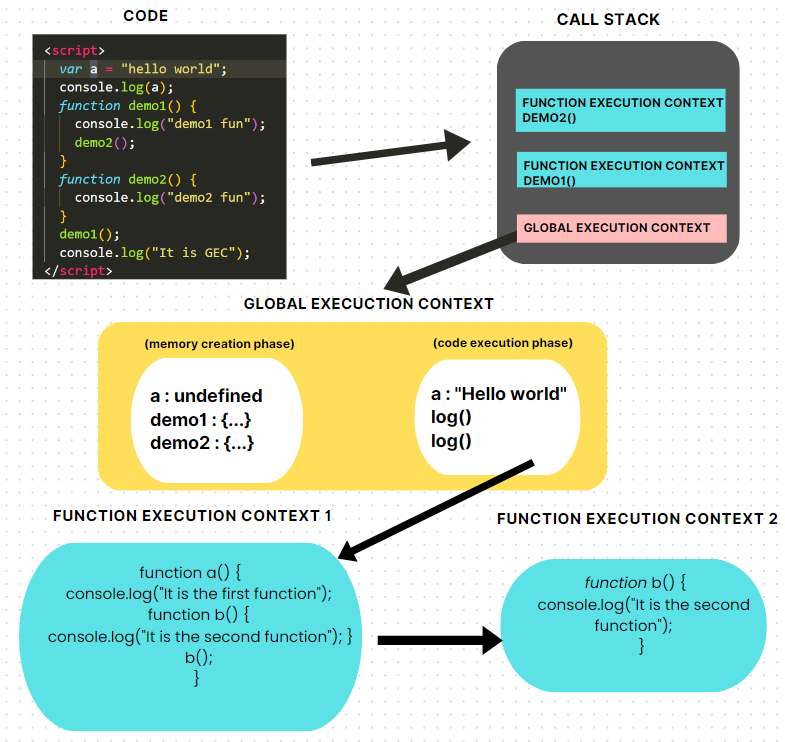
Execution Context
It is an environment which handles the execution of java script code.
- Everything in Java script happens inside the execution context. (Consider it as a big container)
- Java Script is a synchronous single-threaded language , “single-threaded” meaning all the lines are executed one by one i.e one command is executed at a time and “synchronous ” meaning in a specific order.
Execution context are of 2 types
- Global Execution Context (GEC)
- Function Execution Context (FEC)
Global Execution Context –
Whenever a browser encounters a javascript file, it creates a default execution context that contains all the javascript file that is not inside a function. This default or base execution context is known as the Global Execution Context
For every java script , their can be only one GEC
Function Execution Context –
Whenever a function is invoked/executed in javascript, a new execution context is created every time inside GEC. This new execution context is known as Function Execution Context
Since every new function call gets it own FEC, it ca be more than one.
How Java Script Engine Works
Whenever a js engine run , few things happens –
- Global execution context is created inside call stack
- Memory is assigned to all the variable and function present.
- Global object or the window object is created. (By default this object points to window object)
- Scope chain is initialized.
------------> Pre-requisite to know (Source - here)
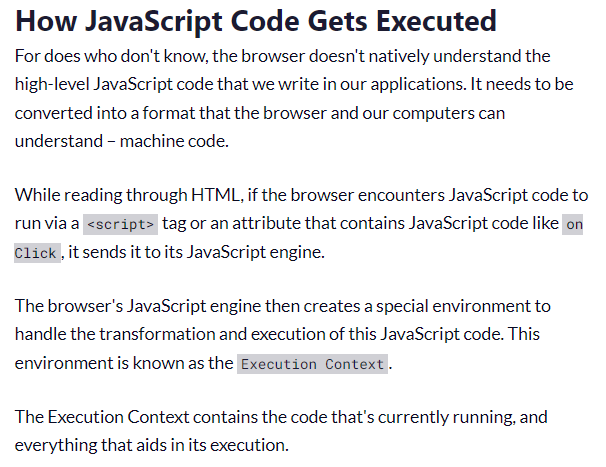
Execution Context has 2 phase –
- Creation Phase
- Execution Phase
1. Creation Phase
Inside the creation phase all the variables are declared as “undefined” and all the functions declaration are stored in the heap memory. This means the variable and function are already made accessible in the current execution context (which is GEC in this case) before the code execution. This concept is known as hoisting.
Memory Component | Code Component |
---|---|
Also know as “Variable Component” | Also know as “Thread of Execution” |
It hold all the variables and functions as “key :value pair” ex : a =10; b = fuc {…} | Here code is executed one by one in a synchronous single-threaded manner. |
2. Execution Phase
Now in this phase, the whole code will again be executed from top to bottom line by line.
- Now as soon as it encounter variable, it will assign the value to it.
- As soon as it finds the function , it’ll create a brand new function execution context on the top of global execution context and function will be executed their.
NOTE : Every time it finds a new java script function , a new function execution context will be created on the top of old execution content , hence forming a stack , which is known as call stack. - As soon as the function is completed, the execution context will be removed in a LIFO manner.
- And at last , the global execution context will also be removed, hence the call stack becomes empty and programs completes.
Conclusion
Whenever a java script files is executed, execution context is created. All the compilation and execution of a java script code happens inside the execution content itself.
Few question which interviewer might ask –
- What is execution context?
- How js engine works behind the scene?
- What is hoisting ?
- What is call stack and how it helps in execution ?
- Can we create more than 1 GEC ?
- Can we create more than 1 FEC ?
Try the above question and comment below your answers .
Read this (recommended) – freecodecamp