What is React
- It is a javascript library managed by Facebook. Remember – It is not a framework.
- React is component-based meaning everything you create is a component.
Eg – A website has <NavBar/> Component , <logo /> component , <Container /> component and <Footer /> component. Create these components individually in react and later use them to create a complete website. Also , the component can be used anywhere else making it reusable.
Quick Prerequisites
- Basics of HTML, CSS, and JavaScript (with ES6). That’s it!
- Install node js to your local computer. Click here
- Any code editor installed on your computer. My recommendation – here
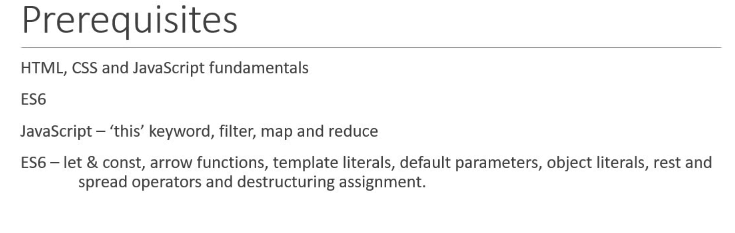
Create your first react project
- Create a folder
- Go inside the folder, right-click, and open Powershell.
- Now write the below command to create react app
npx create-react-app hello-world cd hello-word npm start
Great! Your first project is running at http://localhost:3000.
Components in react
- Any logic/function can be made into a component in react.
- Component is reusable
- Main Component – <App/>, rest are child component and is wrapped inside the main component.
2 types of components –
Functional Component | Class Component |
---|---|
Normal javascript function | render() method compulsory |
function Title() { return <h1>I am Title</h1>; } | class Title extends React. Component { render(){ return <h1>I am Title</h1>; } } |
Class vs Function Component (code difference)
LearnClassComponent.js
---------------------
import React from "react";
class LearnClass extends React.Component {
render() {
return (
<>
<h2>Hello, I am class component </h2>
</>
);
}
}
export default LearnClass;
LearnFunctionComponent.js
-----------------------
const LearnFunction = () => {
return (
<>
<h3>I am function component</h3>
</>
);
};
export default LearnFunction;
Finally, render the above component into <App /> Component
App component can also be written in either class component or function component
App.js (Class comp.)
---------------------
import React from "react";
import LearnClass from "./Component/LearnClassComponent";
import LearnFunction from "./Component/LearnFunctionComponent";
class App extends React.Component {
render() {
return (
<>
<h1>hello</h1>
<LearnClass />
<LearnFunction />
</>
);
}
}
export default App;
App.js (Function comp.)
-----------------------
import LearnClass from "./Component/LearnClassComponent";
import LearnFunction from "./Component/LearnFunctionComponent";
function App() {
return (
<>
<h1>hello</h1>
<LearnClass />
<LearnFunction />
</>
);
}
export default App;
Note - Try to use functional component as much as possible
JSX –
- JSX provides you to write HTML/XML-like structures (e.g., DOM-like tree structures) in the same file where you write JavaScript code, then the preprocessor or transpiler (i.e babel) will transform these expressions into actual JavaScript code
- JSX is just another form of calling React.createElement()
- It is nothing but HTML wrapped inside a syntactical sugar
Syntax -
What you write in react :
<div>Hello WAC</div>
How it is conveted y tranpier so that browerser can read :
React.createElement("div", null, "Hello WAC");
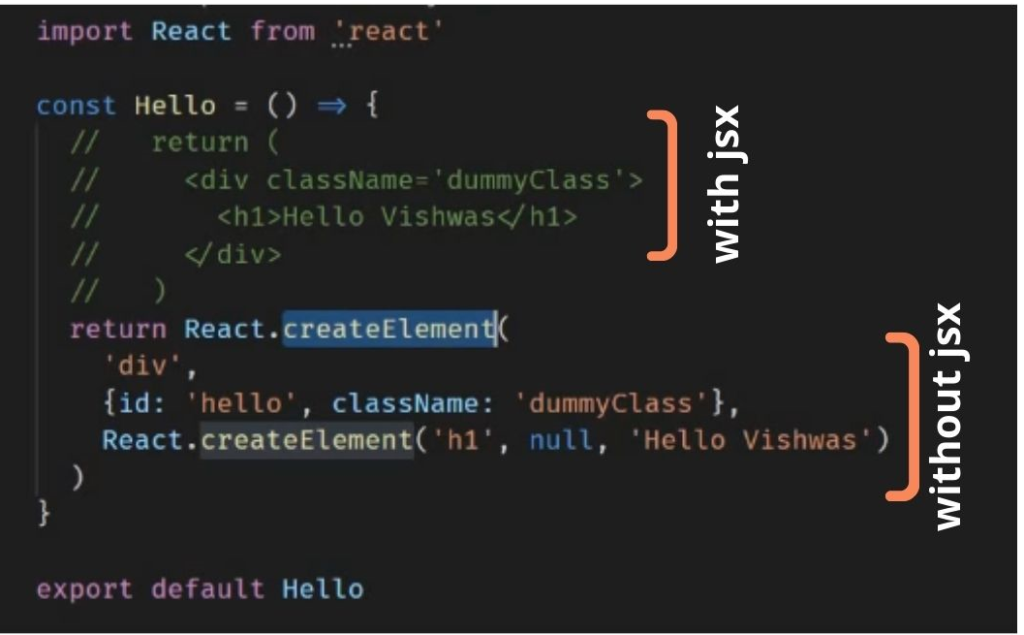
Few differences between normal HTML and JSX
HTML | JSX |
---|---|
– class -for | Some attribute’s name is changed in jsx -className -htmlFor |
onclick tabindex | Camel case events property naming convenction onClick tabIndex |
Props –
- It’s an optional property that can be passed within the component
- It helps in making components dynamic
LearnFunctionComponent.jsx -: ---------------------------- const LearnFunction = (props) => { console.log(props); return ( <> <h3> Hello {props.name} a.k.a. {props.heroName} </h3> </> ); }; export default LearnFunction; App.jsx - : -------- import React from "react"; import LearnFunction from "./Component/LearnFunctionComponent"; class App extends React.Component { render() { return ( <> <LearnFunction name="Clark" heroName="Superman" /> <LearnFunction name="Dyna" heroName="Wonder Women" /> </> ); } } export default App;
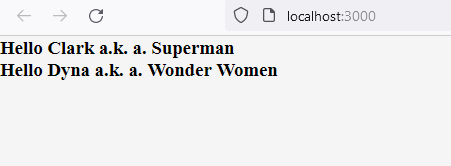
In the above example , inside <App/> component , “name ” and “heroName” are passed as a attribute prop to the <LearnFunction/> component . Inside LearnFunction.jsx file, we are taking those attributes as a prop and using them according to our requirement
Children Props
Sometimes we don’t know what will the content of a prop, in that case, prop. children become handy
LearnFunction.jsx - ------------------ const LearnFunction = (props) => { console.log(props); return ( <> <h3> Hello {props.name} a.k. a. {props.heroName} </h3> {props.children} //can access any data sent as an child prop </> ); }; export default LearnFunction; App.jsx- ------------------ import React from "react"; import LearnFunction from "./Component/LearnFunctionComponent"; class App extends React.Component { render() { return ( <> <LearnFunction name="Clark" heroName="Superman" /> <LearnFunction name="Dyna" heroName="Wonder Women" /> <LearnFunction> <button>Release Now</button> //send an an child component , since weapd inside component </LearnFunction> </> ); } } export default App;
Props in Class Component
In the class component, to access props, we need to write “this.props” instead of just “props”
LearnClass.jsx
-----------------
import React from "react";
class LearnClass extends React.Component {
render() {
// console.log(this.props);
return (
<>
<h2>Hello, {this.props.name} </h2>
<p>{this.props.children}</p>
</>
);
}
}
export default LearnClass;
App.js -
-------
import React from "react";
import LearnClass from "./Component/LearnClassComponent";
class App extends React.Component {
render() {
return (
<>
<LearnClass name="Clark" />
<p>Upcoming movie in 2023</p>
</>
);
}
}
export default App;
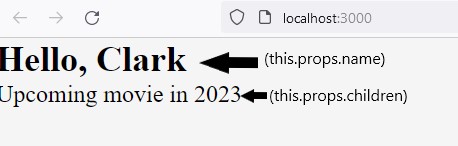
One Drawback of props
suppose in the above example, you want to change the value of props, from “Superman” to “SuperWomen”, you can’t, not because superwomen was not a film, it is just because props are immutable, meaning you cant change or override the value of props.
React Component act as a pure function, when it comes to props
LearnClass.jsx (error)
----------------
import React from "react";
class LearnClass extends React.Component {
render() {
//trying to change props value
this.props.name = "Superwomen"; //not the right way
return (
<>
<h2>Hello, {this.props.name} </h2>
<p>{this.props.children}</p>
</>
);
}
}
export default LearnClass;
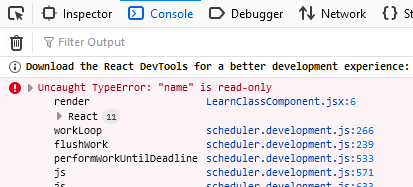
So, to fix this, the state comes into the picture.
State
- The state is a built-in React object that is used to contain data or information about the component
- State value is mutable and can change over time depending upon the user’s action
- State is maintained inside the component
- State influence what is rendered in the browser
State using Class Component
LearnClass.js
--------------
import React from "react";
class LearnClass extends React.Component {
constructor() {
//it is required to call parent class construcotr
super();
//state defined inside constuctor, this is recommended
this.state = { name: "Kent" };
}
changeText = () => {
this.setState({
name: "clark",
});
};
render() {
return (
<>
<h2>Hello, {this.state.name} </h2>
<button id="btn" onClick={() => this.changeText()}>
subscribe
</button>
</>
);
}
}
export default LearnClass;
In the above code, you should always right the state inside the constructor because whenever the component class is created(LearnClass in this case), the constructor is the first method to be invoked, meaning all the values are initialized first.
Initializing State inside the class, not inside the constructor (not recommended)
import React from "react"; class LearnClass extends React.Component { //state defined within class state = { name: "Rahul", roll: 102 }; render() { return ( <> <h3> {this.state.name} , Naam to suna hoga </h3> <p>Aur roll number bhi {this.state.roll}</p> </> ); } } export default LearnClass;
When the state is defined within a class, no need to use “this.state” to define, directly use “state”.
State using Functional Component
Earlier it was told that State can only be used in the Class component. To overcome this issue, react came with hooks, which allow us to use state property in functional components as well.
//LearnFunction.jsx import { useState } from "react"; const LearnFunction = () => { let [count, setCounter] = useState(0); let increaseCounter = () => { setCounter(count + 1); }; return ( <> <h3>Hello {count}</h3> <button onClick={() => setCounter(increaseCounter)}>Increase (+) </button> </> ); }; export default LearnFunction; App.js ------- import React from "react"; import LearnFunction from "./Component/LearnFunctionComponent"; function App() { return ( <> <LearnFunction /> </> ); } export default App;
Props
- Props get passed to the same or other components
- props are immutable
- props are function parameter
- In the function component, we write “props”, whereas in-class component, and we write “this.props”
State
- The state is managed within the component
- state can be changed
- the state is a variable declared inside a function body
- In the functional component, state is used by “hooks” and in class component, “this.state”
For the sake of article length and keeping in mind, that this article is only to make you basic ready in React, I will now conclude this article.
Conclusion
Almost any further concept you learn after this in react, some of the above concepts will definitely come into picture.
So that you are comfortable with above topics, you are free and ready to learn some more important topic in ract
I am listing important concepts which you need to learn in React.
- Hooks
- React Router
- Context API
- Axios
- Redux
Hope this article helped. Thank you for reading.