In this article, you will learn how to apply a basic CRUD operation in React JS.
What is CRUD
Crud stands for
- Create
- Read
- Update
- Delete
No application in the world can be made without using CRUD operation. Let’s say, you uploaded a picture on Facebook, well that is a Create operation. To see how many likes/comments you got, you refresh your feed and see your post is reflecting on your feeds . that is a Read Operation. Soon you realized your caption has a spelling mistake, so you edit and corrected it, that is noting but Update Operation. After a while, you removed the post because you thought life is more than social media 😜 , so you went ahead and deleted the post, that is Delete Operation.
Now that Mark Zuckerberg and you guys, both understood what is crud operation, let’s understand it more with a code.
About the application
We are going to build a simple application where user can
- enter their name and email
- view their name and email
- edit and update their name and email
- delete their name and email
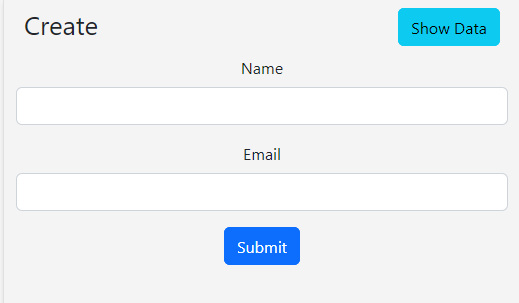
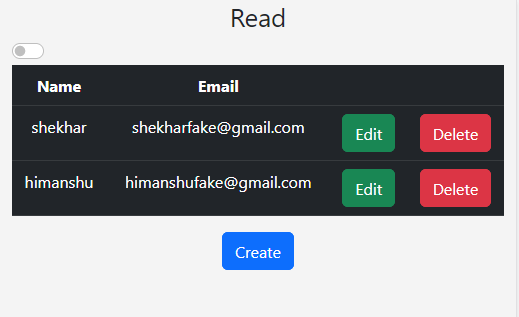
We are going to build the above same application as shown in the image
Wach Video Instead
Let’s build now
Pre-requisite
Basic of HTML, CSS and Java Script
Familier with basics of ES6 feture
Basic of React JS like functional component ,props , states , React Router , useState() , useEffect()
Note - Even you are not very sure of the above topic, you can read the article. I have tried to make it as simple as possible.
Tech stack, packages and other stuff used to build this application
REACT JS , AXIOS (NPM PACKAGE) , BOOTSTRAP , HTML, CSS.
Note - One more thing need to build the application is patience and curiosity(hope you guys have installed that already).
Let’s get the basic react project setup
npx create-react-app CrudProject
We would also need a few node packages for the project, so let’s install those dependencies now itself.
npm i axios
npm i react-router-dom
src/App.js
import "./App.css"; function App() { return ( <div className="container"> <h2>Welcome to CRUD operation</h2> </div> ); } export default App;
Also I have just imported the CSS CDN of bootstrap 5 to make the application simple
public/index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <link rel="icon" href="%PUBLIC_URL%/favicon.ico" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <meta name="theme-color" content="#000000" /> //bootstrap 5 css CDN added <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-0evHe/X+R7YkIZDRvuzKMRqM+OrBnVFBL6DOitfPri4tjfHxaWutUpFmBp4vmVor" crossorigin="anonymous" /> <meta name="description" content="Web site created using create-react-app" /> <link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" /> <link rel="manifest" href="%PUBLIC_URL%/manifest.json" /> <title>React App</title> </head> <body> <noscript>You need to enable JavaScript to run this app.</noscript> <div id="root"></div> </body> </html>
Now that your boilerplate is ready , let’s perform our operations
Also since we are understanding the CRUD operation from the frontend side, I have used mockAPI as a backend server, so make the tutorial point-to-point and beginner-friendly
Create.js (CREATE Operation)
import axios from "axios"; import React, { useState } from "react"; import { useNavigate } from "react-router"; import { Link } from "react-router-dom"; const Create = () => { const [name, setName] = useState(""); const [email, setEmail] = useState(""); const history = useNavigate(); const handleSubmit = (e) => { e.preventDefault(); console.log("clciekd"); axios .post("https://62a59821b9b74f766a3c09a4.mockapi.io/crud-youtube", { name: name, email: email, }) .then(() => { history("/read"); }); }; return ( <> <div className="d-flex justify-content-between m-2"> <h2>Create</h2> <Link to="/read"> <button className="btn btn-primary">Show Data</button> </Link> </div> <form> <div className="mb-3"> <label className="form-label">Name</label> <input type="text" className="form-control" onChange={(e) => setName(e.target.value)} /> </div> <div className="mb-3"> <label className="form-label">Email address</label> <input type="email" className="form-control" aria-describedby="emailHelp" onChange={(e) => setEmail(e.target.value)} /> </div> <button type="submit" className="btn btn-primary" onClick={handleSubmit} > Submit </button> </form> </> ); }; export default Create;
Read.js (Read Operation & Delete Operation)
import React, { useState, useEffect } from "react"; import axios from "axios"; import { Link } from "react-router-dom"; const Read = () => { const [data, setData] = useState([]); const [tabledark, setTableDark] = useState(""); function getData() { axios .get("https://62a59821b9b74f766a3c09a4.mockapi.io/crud-youtube") .then((res) => { setData(res.data); }); } function handleDelete(id) { axios .delete(`https://62a59821b9b74f766a3c09a4.mockapi.io/crud-youtube/${id}`) .then(() => { getData(); }); } const setToLocalStorage = (id, name, email) => { localStorage.setItem("id", id); localStorage.setItem("name", name); localStorage.setItem("email", email); }; useEffect(() => { getData(); }, []); return ( <> <div className="form-check form-switch"> <input className="form-check-input" type="checkbox" onClick={() => { if (tabledark === "table-dark") setTableDark(""); else setTableDark("table-dark"); }} /> </div> <div className="d-flex justify-content-between m-2"> <h2>Read Operation</h2> <Link to="/"> <button className="btn btn-secondary">Create</button> </Link> </div> <table className={`table ${tabledark}`}> <thead> <tr> <th scope="col">#</th> <th scope="col">Name</th> <th scope="col">Email</th> <th scope="col"></th> <th scope="col"></th> </tr> </thead> {data.map((eachData) => { return ( <> <tbody> <tr> <th scope="row">{eachData.id}</th> <td>{eachData.name}</td> <td>{eachData.email}</td> <td> <Link to="/update"> <button className="btn-success" onClick={() => setToLocalStorage( eachData.id, eachData.name, eachData.email ) } > Edit{" "} </button> </Link> </td> <td> <button className="btn-danger" onClick={() => handleDelete(eachData.id)} > Delete </button> </td> </tr> </tbody> </> ); })} </table> </> ); }; export default Read;
Update.js (Update Operation)
import axios from "axios"; import React, { useEffect, useState } from "react"; import { Link, useNavigate } from "react-router-dom"; const Update = () => { const [id, setId] = useState(0); const [name, setName] = useState(""); const [email, setEmail] = useState(""); const navigate = useNavigate(); useEffect(() => { setId(localStorage.getItem("id")); setName(localStorage.getItem("name")); setEmail(localStorage.getItem("email")); }, []); const handleUpdate = (e) => { e.preventDefault(); console.log("Id...", id); axios .put(`https://62a59821b9b74f766a3c09a4.mockapi.io/crud-youtube/${id}`, { name: name, email: email, }) .then(() => { navigate("/read"); }); }; return ( <> <h2>Update</h2> <form> <div className="mb-3"> <label className="form-label">Name</label> <input type="text" className="form-control" value={name} onChange={(e) => setName(e.target.value)} /> </div> <div className="mb-3"> <label className="form-label">Email address</label> <input type="email" className="form-control" value={email} onChange={(e) => setEmail(e.target.value)} /> </div> <button type="submit" className="btn btn-primary mx-2" onClick={handleUpdate} > Update </button> <Link to="/read"> <button className="btn btn-secondary mx-2"> Back </button> </Link> </form> </> ); }; export default Update;
App.js
import "./App.css"; import { BrowserRouter, Routes, Route } from "react-router-dom"; import Create from "./Components/Create"; import Read from "./Components/Read"; import Update from "./Components/Update"; function App() { return ( <div className="container"> <BrowserRouter> <Routes> <Route exact path="/" element={<Create />}></Route> <Route path="/read" element={<Read />}></Route> <Route path="/update" element={<Update />}></Route> </Routes> </BrowserRouter> </div> ); } export default App;
Conclusion
In the above article , you have understood how to apply crud operation using react js . We have also seen how axios package can help in handling API .
Hope you guys like the article.
react crud project
Sir Good Afternoon, plz send me source code
Really appreciate sir and thank you. . I learn good things .